Phyton Quiz with Multiple Choice
Take this Python quiz to test your knowledge of Python programming language. Answer multiple-choice questions on topics like syntax, functions, data types, and more. This Python quiz features multiple-choice questions on a variety of topics, including syntax, functions, data types, and more. Test your knowledge of Python programming language and see how you measure up!
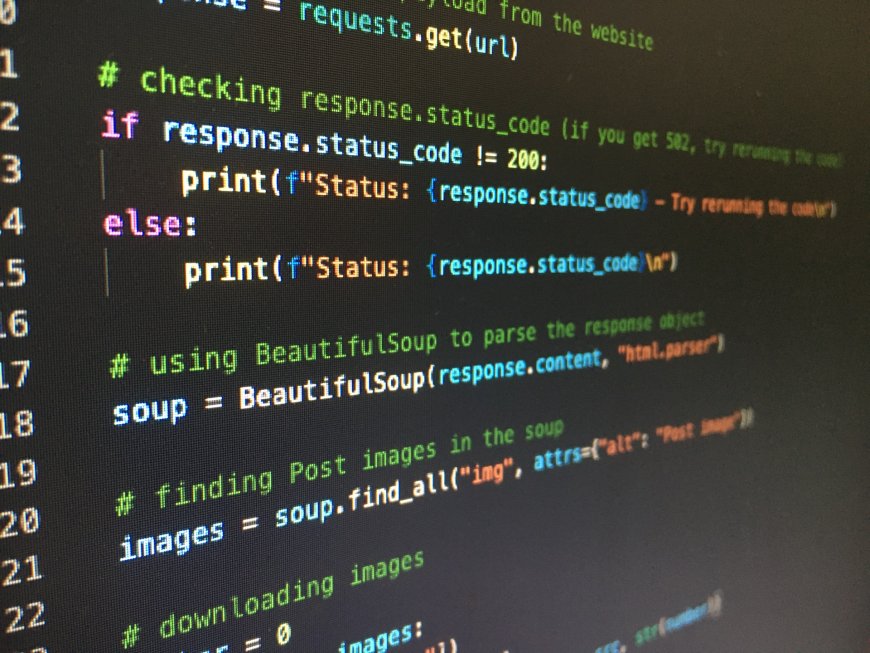
What's Your Reaction?
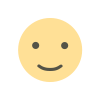
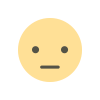
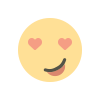
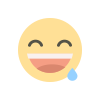
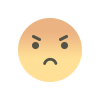
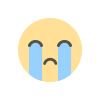
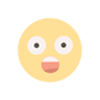